@alemauro
The default for Canvas is to limit the width of the page except on certain pages like outcomes and the gradebook. Here is the actual CSS rule that is contained within Canvas. You can tell that the maximum width is set to 1366 pixels.
body:not(.full-width):not(.outcomes) .ic-Layout-wrapper {
max-width: 1366px;
}
If you are seeing full screen, it is because your institution has overridden the defaults. This can be done through custom CSS or custom JavaScript by the Canvas Administrator for that institution.
JavaScript
When I look at the Cornell site that you have listed, they have added a classname of "full-width" to the body. That can be done using JavaScript.
The default classes for one of my pages is
class="with-left-side course-menu-expanded padless-content pages primary-nav-expanded context-course_896851 responsive_admin_settings responsive_awareness responsive_misc show webkit chrome no-touch"
If you add "full-width" to that, it make no longer has the max-width CSS attribute.
class="full-width with-left-side course-menu-expanded padless-content pages primary-nav-expanded context-course_896851 responsive_admin_settings responsive_awareness responsive_misc show webkit chrome no-touch"
Here is the JavaScript needed to make the change. You would pick exactly one of these lines: the first one to make it go full width or the second one to make it not go full width.
document.body.classList.add('full-width');
document.body.classList.remove('full-width');
You would probably want to incorporate logic to limit which pages that should happen with. You probably want the gradebook to be full screen.
CSS
If your institution wants to remove the 1366px limitation on all pages, they need to override the Canvas CSS. This is done by increasing the specificity (putting additional selectors in) or using !important at the end. Here is the rule I use.
body:not(.full-width):not(.outcomes) #wrapper.ic-Layout-wrapper {max-width:none;}
This has an extra selector "#wrapper.ic-Layout-wrapper" than the Canvas rule that sets "max-width: 1366px;" so it is more specific and will get implemented instead of Canvas'.
The institution would not need to do anything to keep the 1366px limitation, it's what Canvas already has. As I said, this is the default.
What can I do?
Everything I've written so far can be done at the institutional level. What about people who don't want to see the full-width on every page? Is there anything they can do?
The answer is yes, but you will need something to insert JavaScript or CSS into the browser. I use Tampermonkey, which is a userscript manager. It allows you to insert JavaScript into the browser like it was part of the content delivered with Canvas.
Tampermonkey doesn't insert CSS directly (it's possible to insert through JavaScript).
The first thing you need to do is to determine what technique your institution is using. Cornell is using the full-width class, another school may override the CSS. Fixing the wrong problem won't help.
To do this (I'm using Chrome or Firefox), go to a content page and press F12. Then switch to the Elements tab and find the body element. This is what Cornell's site looks like in Chrome. Note the full-width classname.
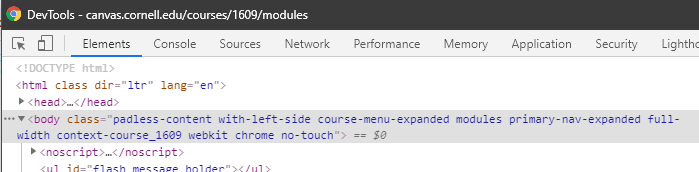
If the full-width classname is not there, then it is likely that they used CSS to override. Removing the class full-width Removing the full-width when it is there is more problematic through CSS. That rule has a ":not(.full-width)" selector, so it doesn't get used when the body contains the full-width class. You would need to override their CSS override to set it back to normal. That means finding their modified rule and then making sure that yours to set it back to 1366px is used. There are a couple of ways to do that: you can make your selector have a higher specificity or you can make sure that yours is loaded after theirs. This is explained in section 6 Cascading in the CSS Cascading and Inheritance Level 3 recommendation.
Then you have to write some JavaScript code that will undo what the institution has done.
This may not be as easy as it sounds. I gave you one line of code that would remove the full-width class from the body. The problem is one of timing. Stuff that you do with Tampermonkey may get executed before the code that your school runs. That is, you may remove the full-width class before it's there, then your institution may come along and put it in there. In other words, it will look like nothing happens. The fix to that is to use mutation observers and watch what happens to the body. Then when the full-width class gets added, you could remove it.
Another issue is deciding what pages you want to remove the full-width from. If you are lucky, your code will execute before your institution's code. Then you could see if the full-width class was already there. For example, when I load the gradebook page, here are my classes that come delivered as part of the HTML.
class="gradebook full-width with-left-side grades primary-nav-expanded context-course_896851 responsive_admin_settings responsive_awareness responsive_misc"
If the full-width is there in the HTML delivered from the page (you can check this with Ctrl-U), then there is no need to add the mutation observer to watch for it. However, it may be that your institution's code is executed before yours, so you may not be that simple, either.
You would then need to figure out what pages you wanted to make full screen. If it's just the content pages, that's relatively easy. But it may also be the quizzes and other things that you want to restore.
You can use window.location.pathname to get the name of the page you're on. I use regular expressions to see where I'm at, but other JavaScript programmers use different approaches.
For example, this code will look for pages or quizzes that have the full-width class on the body and remove the class. Technically, you don't have to check to see if it contains full-width,
if (/^\/courses\/\d+\/(pages|quizzes)/.test(window.location.pathname) &&
document.body.classList.contains('full-width')) {
document.body.classList.remove('full-width');
}
Technically, you don't have to check to see if it contains the full-width class. According to the classList.remove() documentation, "If the string is not in the list, no error is thrown, and nothing happens." That means that you could shorten the code to this:
if (/^\/courses\/\d+\/(pages|quizzes)/.test(window.location.pathname)) {
document.body.classList.remove('full-width');
}
You could also take the exclusion route and say I don't want this to happen on any page beginning with gradebook.
if (!/^\/courses\/\d+\/gradebook/.test(window.location.pathname)) {
document.body.classList.remove('full-width');
}
I still suspect that you will need to attach a mutation observer to the body element.
All of that would go inside a Tampermonkey script and then you use the //include or //match lines to say what pages it should run on. If you are specific enough in the Tampermonkey metadata and when it should run, you could remove the check for the page within the script and just go back to the one line.
I don't know your skill level with programming and we don't know which approach your institution is using, so I could get really lengthy in my response without accomplishing anything.
Easy Way
By far, the easiest (no programming required) way to accomplish what you want is to take your browser out of full screen mode and then drag it so that the extra space isn't on the right side.
Comments
Tampermonkey is a user script manager. Things you do with it only affect your and the browser you have it installed on. It will not affect everyone else at the institution. Changes the institution makes affect everyone.
I actually use Tampermonkey to insert CSS into my page to use the full width. By using CSS, I don't have to worry about mutation observers. I'm of the mindset that I paid extra to get the wide monitor, I can resize my window if I need to, but I hate the wasted space on the side because then I have to do more scrolling.
Canvas decided it's too hard for people to read with really wide pages and so they narrowed the length of each line. The Web Style Guide site provides some research to back that up (although their statistics are dated). However, their arguments about long pages having advantages don't necessarily hold up. Canvas recommends shorter pages and I have found that students (people in general) tend not to read everything when it's really long. The two seem at odds, but you have to think beyond your screen. Some people may be reading the content on a narrow-screen device like a smart phone. If you put a lot of content on the page at full-width mode so students don't have to scroll down, then they have to scroll down all the more on their phones.
This discussion post is outdated and has been archived. Please use the Community question forums and official documentation for the most current and accurate information.