chriscas,
I think using the next sibling selector, +, will help you with the date picker. You could probably also use the general sibling selector, ~, as well.
Try this to disable, but leave visible, the information. It's actually tied to the field so you don't have to worry about specific row numbers in a table.
$('#course_start_at').prop('disabled',true);
$('#course_start_at + button.ui-datepicker-trigger').prop('disabled',true);
if you would to hide the date picker instead of disabling it, then use
$('#course_start_at + button.ui-datepicker-trigger').hide();
Here's what it looks like if I disabled the start date and hide the course picker for it. I left the end date alone to show the contrast.
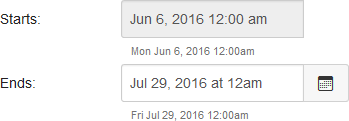
Each of those fields has an id associated with it. They are course_start_at, course_conclude_at, course_course_format. The last one has no date picker. So, if you wanted to change all three, you could do this:
$('#course_start_at').prop('disabled',true);
$('#course_start_at + button.ui-datepicker-trigger').prop('disabled',true);
$('#course_conclude_at').prop('disabled',true);
$('#course_conclude_at + button.ui-datepicker-trigger').prop('disabled',true);
$('#course_course_format').prop('disabled',true);
or use this to disable the dates and hide the datepickers
$('#course_start_at').prop('disabled',true);
$('#course_start_at + button.ui-datepicker-trigger').hide();
$('#course_conclude_at').prop('disabled',true);
$('#course_conclude_at + button.ui-datepicker-trigger').hide();
$('#course_course_format').prop('disabled',true);
Finally, if someone really wants to hide them completely, verses disabling them, here is some JavaScript/jQuery code that will do that without relying on the row number in the table. It uses the jQuery .closest() function, will will traverse up the DOM tree, so I find the field and then work backwards to find the parent table row.
$('#course_start_at').closest('tr').hide();
$('#course_conclude_at').closest('tr').hide();
$('#course_course_format').closest('tr').hide();
A more extendable approach would be to use an array of the fields you want to remove.
var hiddenFields = [ 'course_start_at', 'course_conclude_at', 'course_course_format' ];
for (var i = 0; i < hiddenFields.length; i++)
{
$('#' + hiddenFields[i]).closest('tr').hide();
}
That loop could be modified to disable the elements instead of hiding them. It won't cause irreparable harm (not the same as it's good programming) to attempt to disable an element that doesn't exist. If jQuery can't find, it, it just won't act on it.
This will disable all of the dates and date pickers as well as the course format.
var hiddenFields = [ 'course_start_at', 'course_conclude_at', 'course_course_format' ];
for (var i = 0; i < hiddenFields.length; i++)
{
$('#' + hiddenFields[i]).prop('disabled', true);
$('#' + hiddenFields[i] + ' + button.ui-datepicker-trigger').prop('disabled', true);
}
This will disable all of the dates and course format and hide the date pickers.
var hiddenFields = [ 'course_start_at', 'course_conclude_at', 'course_course_format' ];
for (var i = 0; i < hiddenFields.length; i++)
{
$('#' + hiddenFields[i]).prop('disabled', true);
$('#' + hiddenFields[i] + ' + button.ui-datepicker-trigger').hide();
}
Of course, all of that is still contained within whatever code you want to use to make sure you're on the right page and the right people have access to it.
I hope that helps. I know I provided lots of ways to accomplish things and that makes it look like a really long solution when it's just a bunch of short ones that someone should pick from, depending on their needs